GitHub Actions for CI-CD: Automating C++ Builds and Deployments
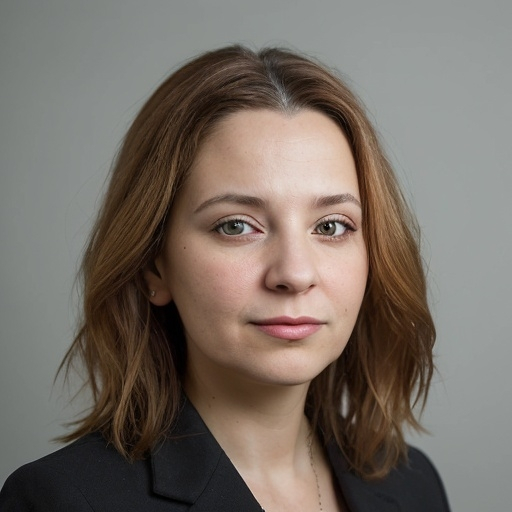

Streamline Your C++ Workflow with GitHub Actions: From Build to Deployment
GitHub Actions is a powerful tool that allows you to automate your development workflow, including building, testing, and deploying your C++ projects. This guide will walk you through setting up a basic GitHub Actions workflow for CI/CD (Continuous Integration and Continuous Deployment), ensuring your C++ projects are built, tested, and deployed seamlessly.
1. Defining Your Workflow
First, navigate to your C++ repository on GitHub and click on "Actions." You'll see a list of suggested workflows, but for now, click on "set up a workflow yourself." GitHub will create a .github/workflows/main.yml
file in your repository. This file defines the actions you want to perform. Here's a basic workflow example:
name: CI
on:
push:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Build
run: |
mkdir build
cd build
cmake ..
make
In this workflow:
- name: Defines the workflow's name.
- on: Specifies the events that trigger the workflow. In this case, it runs on every push to the 'main' branch.
- jobs: Defines the set of tasks to be executed. Here, we have a single job named 'build'.
- runs-on: Specifies the operating system where the workflow will run. 'ubuntu-latest' is a common choice for C++ builds.
- steps: A sequence of actions to be performed in the job. We first use the
actions/checkout@v2
action to check out your repository code. Then, we use therun
step to execute a series of commands to build your C++ project.
2. Building Your C++ Project
The 'run' step executes the commands you define. In our example, we:
- Create a
build
directory. - Change to the
build
directory. - Use
cmake
to configure your build system. - Use
make
to build your project.
You can modify the build steps based on your specific project requirements. For instance, you may use a different build system like qmake
or scons
.
3. Adding Tests and Deployment (Optional)
Once your project is built, you can add further steps to test your code and deploy it.
- Testing: You can include steps to run unit tests using tools like
gtest
orCatch2
. - Deployment: For deployment, you can use the following approaches:
- Manual: After a successful build and test, the workflow can notify developers or send artifacts for manual deployment.
- Automated: You can use actions like
actions/upload-artifact@v2
to upload build artifacts to GitHub, then utilize tools likedocker
oraws-cli
to deploy the artifacts to a server or cloud platform.
Here's an example of how to include testing and manual deployment:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Build
run: |
mkdir build
cd build
cmake ..
make
- name: Run Tests
run: |
cd build
ctest -j 4
- name: Upload Artifacts
uses: actions/upload-artifact@v2
with:
name: build-artifacts
path: build/
This code adds a step to run tests with ctest
, and another step to upload the build artifacts to GitHub. You can then manually download these artifacts and deploy them to your desired environment.
4. Advanced Configurations
- Workflow Triggers: You can trigger workflows on various events, like pull requests, releases, or scheduled events using the
on
section in your.yml
file. - Secrets: For sensitive information like API keys, you can use GitHub Secrets. These are encrypted variables stored within your repository settings.
- Environment Variables: Use environment variables to manage configurations within your workflows.
- Caching: Speed up your builds by caching dependencies using the
actions/cache@v2
action.
5. Key Takeaways
GitHub Actions provides a powerful way to automate your C++ development process. By implementing workflows for building, testing, and deploying, you can enhance your productivity and code quality. This guide offers a starting point for automating your C++ workflow. Explore the comprehensive GitHub Actions documentation for advanced features and customize your workflows to fit your specific needs.
Remember: Continuous integration and deployment are essential for modern software development practices. GitHub Actions makes it easy to streamline your C++ workflow and achieve faster and more reliable releases.