Automating Java Projects with GitHub CI-CD Pipelines
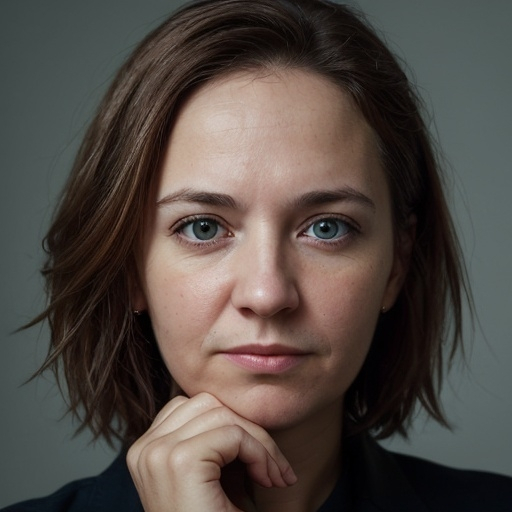

In the fast-paced world of software development, efficiency and reliability are paramount. One powerful tool to achieve these goals is Continuous Integration/Continuous Delivery (CI/CD), and GitHub Actions provides an exceptional platform to implement it for your Java projects. This guide will walk you through setting up a robust CI/CD pipeline within your GitHub repository, empowering you to automate builds, tests, and deployments.
Setting Up Your GitHub Workflow
-
Create a
.github/workflows
Directory: Within your Java project's repository, create a directory named.github/workflows
. This is where you'll store your workflow files, which define the steps in your CI/CD pipeline. -
Define Your Workflow File: Within the
.github/workflows
directory, create a YAML file (e.g.,ci.yml
). This file will outline the stages and jobs of your CI/CD pipeline.
name: Java CI
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v3
- name: Set up Java
uses: actions/setup-java@v3
with:
java-version: '11'
- name: Build with Maven
run: mvn clean package
Explanation:
name
: Provides a human-readable name for your workflow.on
: Specifies triggers for the workflow. In this example, it runs on pushes to themain
branch and pull requests targetingmain
.jobs
: Defines the individual tasks within your workflow. Here, we have abuild
job.runs-on
: Specifies the operating system runner for the job. We're using the latest Ubuntu distribution.steps
: A list of actions to perform within the job.
Enhancing Your CI/CD Pipeline
1. Running Tests:
- name: Run Tests
run: mvn test
This step executes your unit tests using Maven. You can customize this command to match your project's testing framework (e.g., JUnit, TestNG).
2. Code Analysis:
- name: Code Analysis
uses: actions/code-analysis@v1
Utilize code analysis tools like SonarQube to identify potential issues in your code.
3. Deploying to Production:
jobs:
deploy:
runs-on: ubuntu-latest
needs: build
steps:
- name: Checkout code
uses: actions/checkout@v3
- name: Deploy to AWS
uses: aws-actions/configure-aws-credentials@v1
with:
aws-access-key-id: ${{ secrets.AWS_ACCESS_KEY_ID }}
aws-secret-access-key: ${{ secrets.AWS_SECRET_ACCESS_KEY }}
- name: Deploy to EC2 instance
run: aws s3 sync target/my-app.jar s3://my-app-bucket/
needs
: Indicates that thedeploy
job depends on the successful completion of thebuild
job.aws-actions/configure-aws-credentials
: Sets up AWS credentials for deployment. ReplaceAWS_ACCESS_KEY_ID
andAWS_SECRET_ACCESS_KEY
with your actual AWS credentials stored as secrets in your repository.run
: Executes deployment commands. This example deploys to an S3 bucket.
Important Considerations:
- Secrets Management: Never commit sensitive information like API keys, passwords, or AWS credentials directly to your repository. Use GitHub Secrets to securely store them.
- Caching: Use caching to speed up your workflow by avoiding redundant downloads of dependencies.
- Testing Strategies: Employ unit tests, integration tests, and end-to-end tests to ensure the quality of your codebase.
Conclusion
Automating your Java projects with GitHub CI/CD pipelines empowers you to streamline development, enhance code quality, and deliver software more efficiently. By leveraging GitHub Actions, you can easily implement a robust pipeline that encompasses building, testing, and deployment, ultimately contributing to a more productive and reliable development process.