Deploying Go Applications with Docker: A Comprehensive Tutorial
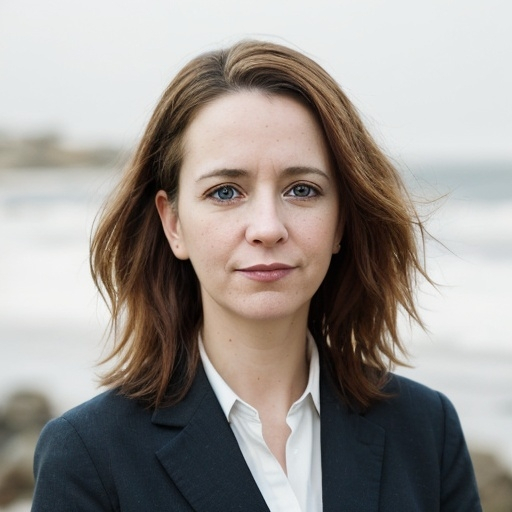

Go, or Golang, is a popular compiled programming language known for its speed, concurrency, and simplicity. Docker, a leading containerization platform, offers a way to package and run Go applications in isolated environments, ensuring consistent performance and deployment across different systems. This guide will walk you through the process of deploying Go applications with Docker, from building your first Dockerfile to deploying your application in a production environment.
Setting Up Your Development Environment
Before we delve into Docker, ensure you have the following prerequisites in place:
- Go Installation: Download and install the latest version of Go from the official website (https://golang.org/). Follow the installation instructions for your operating system.
- Docker Installation: Docker Desktop is a convenient way to install and manage Docker on Windows, macOS, and Linux. You can find the download links at https://www.docker.com/products/docker-desktop.
Creating a Simple Go Application
For demonstration purposes, let's build a basic web server using Go:
import ( "fmt" "net/http" )
func main() { http.HandleFunc("/", func(w http.ResponseWriter, r
*http.Request) { fmt.Fprintf(w, "Hello from Go!\n") })
fmt.Println("Server listening on port 8080")
http.ListenAndServe(":8080", nil)
} ```
Save this code as `main.go`. You can run this application directly using the
command `go run main.go` and access it through your browser at
`http://localhost:8080`.
## Dockerizing Your Go Application
Now, let's create a Dockerfile to package our Go application:
```dockerfile FROM golang:1.19-alpine AS builder
WORKDIR /app
COPY . .
RUN go mod download && go build -o main .
FROM alpine:3.16
WORKDIR /app
COPY --from=builder /app/main .
EXPOSE 8080
CMD ["/app/main"] ```
**Explanation:**
* **`FROM golang:1.19-alpine AS builder`:** We start with a base image, `golang:1.19-alpine`, which provides the necessary Go runtime and tools. We use the `AS builder` keyword to create an intermediate build stage.
* **`WORKDIR /app`:** Sets the working directory within the Docker container to `/app`.
* **`COPY . .`:** Copies all files from the current directory to the container's working directory.
* **`RUN go mod download&& go build -o main .`:** Downloads Go dependencies and builds the application.
* **`FROM alpine:3.16`:** We create a new stage based on the `alpine:3.16` image, which is a lightweight Linux distribution.
* **`COPY --from=builder /app/main .`:** Copies the built `main` executable from the previous `builder` stage.
* **`EXPOSE 8080`:** Exposes port 8080 within the container.
* **`CMD ["/app/main"]`:** Sets the command to execute when the container starts.
## Building and Running Your Docker Image
To build the Docker image, navigate to the directory containing your
`Dockerfile` and run:
`bash docker build -t my-go-app .`
Replace `my-go-app` with your desired image name. This will build the image
and tag it with the name `my-go-app`.
Now, run the container using:
`bash docker run -p 8080:8080 my-go-app`
This command maps port 8080 on your host machine to the container's port 8080.
You can now access your Go application at `http://localhost:8080` in your
browser.
## Optimizing Your Docker Image
To make your Docker image more efficient, consider the following:
* **Multi-stage builds:** Use multi-stage builds (as demonstrated in the example) to separate build dependencies from the final runtime image, reducing image size.
* **Alpine Linux:** Use Alpine Linux as a base image for its small size.
* **Minimal dependencies:** Only include the necessary Go packages and libraries in your application.
* **Static linking:** Use static linking to bundle all dependencies into your executable, making it more portable.
## Deploying Your Go Application with Docker
There are various ways to deploy your Dockerized Go application:
* **Docker Hub:** Push your image to Docker Hub (<https://hub.docker.com/>) for easy sharing and distribution.
* **Kubernetes:** Deploy your application to a Kubernetes cluster for automatic scaling, load balancing, and high availability.
* **Docker Compose:** Use Docker Compose to manage multiple containers and their dependencies for a more complex application.
* **Cloud platforms:** Deploy your application to cloud providers such as AWS, Azure, or Google Cloud for scalability and ease of management.
## Conclusion
By following this guide, you have learned the fundamentals of deploying Go
applications using Docker. From building a Dockerfile to optimizing your image
and deploying your application, this tutorial equips you with the knowledge to
effectively containerize your Go projects. Remember to explore Docker's
features and best practices to enhance your workflow and create robust and
efficient deployments.