Improving MongoDB Read Performance: Techniques for Faster Data Retrieval
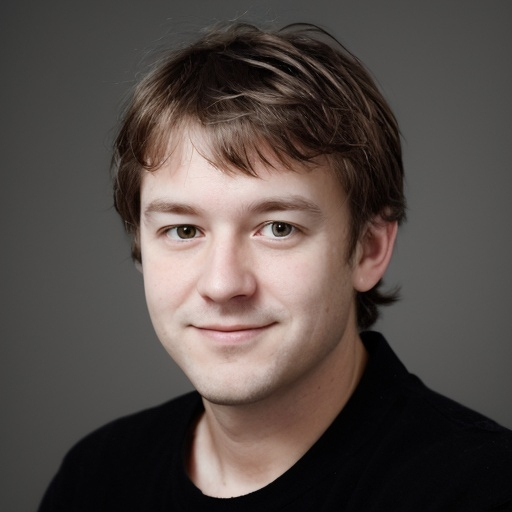

MongoDB is a powerful NoSQL database known for its flexibility and scalability. However, as your data grows, you might encounter performance bottlenecks, particularly with read operations. To ensure smooth and efficient data retrieval, optimizing MongoDB read performance is crucial. This guide explores various techniques to achieve faster data access in your MongoDB applications.
1. Indexing: The Foundation of Efficient Queries
Indexing is the cornerstone of efficient MongoDB read operations. By creating indexes on frequently queried fields, you enable MongoDB to quickly locate relevant documents without scanning the entire collection.
- Choose the Right Index Types: MongoDB offers different index types, each suited for specific use cases. Utilize single-field indexes for simple queries, compound indexes for multi-field searches, and text indexes for full-text searches.
- Optimize Index Selection: Carefully consider which fields to index based on your most common query patterns. Avoid indexing fields that are rarely used in queries.
- Utilize Unique Indexes: For fields that must be unique (e.g., primary keys), create unique indexes to enforce data integrity and enhance lookup operations.
2. Query Optimization: Writing Efficient Queries
Efficient query writing plays a crucial role in minimizing read times. Here's how to craft queries for optimal performance:
- Limit the Number of Fields Returned: Specify only the fields you need using projection. Retrieving unnecessary data can significantly impact performance.
- Use Efficient Query Operators: Familiarize yourself with MongoDB's query operators and their performance implications. For instance, the
$in
operator is generally faster than multiple$eq
operators. - Avoid Deeply Nested Queries: Deeply nested queries can be computationally expensive. Simplify your queries by restructuring data or utilizing aggregation pipelines.
3. Data Modeling: Structuring for Read Optimization
Data modeling plays a significant role in read performance. Consider these strategies:
- Embed Related Data: Embed related data within a document to minimize joins and improve read speed.
- Use Sharding for Scalability: For large datasets, sharding distributes data across multiple servers, improving read performance by parallelizing queries.
- Denormalization: Carefully consider denormalizing your data structure to avoid unnecessary joins and increase read efficiency.
4. Server Configuration: Tuning for Read Performance
Configuration settings on your MongoDB server directly impact read performance. Here are some key considerations:
- Memory Allocation: Allocate sufficient memory to MongoDB to cache frequently accessed data in RAM, reducing disk I/O operations.
- WiredTiger Engine: For optimal read performance, leverage the WiredTiger storage engine, which offers features like caching and efficient data retrieval.
- Connection Pooling: Utilize connection pooling to reduce the overhead of establishing new connections for each read operation.
5. Monitoring and Profiling: Identifying Bottlenecks
Continuous monitoring and profiling are essential to identify and address performance bottlenecks.
- Use MongoDB's Monitoring Tools: Utilize MongoDB's built-in monitoring tools to track query performance, index usage, and other metrics.
- Profiling Queries: Enable query profiling to gather detailed information about query execution times and analyze areas for optimization.
6. Caching: Accelerating Data Retrieval
Caching is a powerful technique for improving read performance by storing frequently accessed data in memory.
- Implement Client-Side Caching: Utilize client-side libraries to cache data locally, reducing the number of database queries.
- Utilize Server-Side Caching: MongoDB supports server-side caching with WiredTiger, enabling the storage of recently accessed data for faster retrieval.
Conclusion
Optimizing MongoDB read performance requires a holistic approach, encompassing indexing, query optimization, data modeling, server configuration, monitoring, and caching. By implementing these techniques, you can significantly enhance the speed and efficiency of data retrieval, ensuring a smooth and responsive experience for your MongoDB applications.